In this post I am continuing my series of Angular articles by showing how to set up two way binding between input elements and model.
Previously I showed how to create a recursive treeview, but in this post I will show how to build interactive user interfaces.
The sample project for this exercise is a simple contact list form where the user is able to add and delete contacts from the list.
The first order of business is to create a new Angular component for the contact list. As you can see from the code below the component defines two methods – removeContact and addContact. Both methods operate on an internal array of contacts.
As you can see from the code, I am using TypeScript to model the contact list logic.
The next step is to bind the component class to the view.
Angular has updated the binding syntax, so there are a few new concepts to cover.
Ng-repeat has been replaced with a new *for loop. However the concept is still the same in that the backing array is bound to the view and additions and removals from the array are automatically reflected in the view.
The next thing to notice is the new (click) binding notation for the button events. Angular 1.x developers may find this new syntax a bit odd, but notice the big improvements in how arguments are passed to the handlers. Argument passing to event handlers in views was very confusing in Angular 1.x and I am very pleased to see that Angular now has simplified this greatly.
Local references to input elements are created using the new #[name] notation. I personally like this style of element naming since the same names are available throughout the view. Notice that the values from the textboxes are available through the value property. The #[name] references are essentially local variables defined in the view.
Finally I have included the model class used to define a contact
I have also included a screenshot of an unstyled version of the contact list.
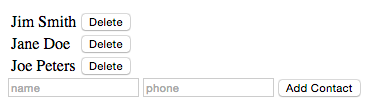
There has been a lot of debate about the new binding syntax, but personally I have started to really like it. It's a big syntactical change from Angular 1.x, but conceptually it's not that different at the end of the day...
Here is a live demo.
The code is also available on GitHub, so feel free to check it out.