I have started exploring Aurelia and figured building a treeview would be a good way to learn more about the framework. The following post is a write up of how I built my treeview.
There seems to be a community forming behind Aurelia, so I figured I'd give it a try and build something of manageable complexity. I landed on a treeview component since it requires me to touch upon several of the different binding types in addition to recursive templates. The treeview supports recursive rendering of a few U.S. states with cities and boroughs. There is also support for expand/collapse of nodes with children.
Aurelia is a modern framework, with support for ES6 out of the box, which requires some setup to get transpiling and the rest of the development environment up and running. In order to simplify the process I recommend downloading the provided skeleton project and use it as a baseline. Aurelia is still a new framework, but the documentation on their website is already impressive and answered most of my questions.
I am no stranger to web frameworks, but the skeleton project is a huge help when learning about Aurelia since it covers several typical SPA scenarios. Also, I was very impressed with the simple and intuitive data binding model offered by the framework.
For the purposes of my treeview I decided to model it as two custom templates; tree-view and tree-node. The components are bound to a decoupled view model which promotes reuse and simplifies unit testing. I am big fan of keeping my view models free of framework dependencies since it makes my code much more portable. This is important since we live in a time where new frameworks are popping up all over the place :-)
Anyway, here's the code:
JavaScript
node-model.js
tree-view.js
tree-node.js
Html
tree-view.html
tree-node.html
main-page.html
As you can tell, the code is relatively simple. At a high level it boils down to two templates with a shared recursive object model. The tree-node component is recursive since it calls itself as part of the template. Below is a screenshot of an unstyled version of the treeview:
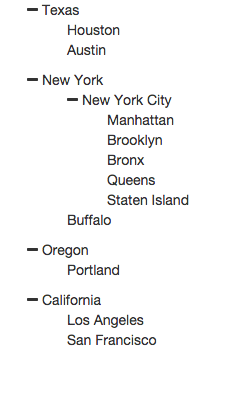