I am continuing my Angular article series and today I want to show you how to integrate non Angular code in Angular. Specifically I will be integrating an existing React/Flux component with an Angular host component.
The React component I am using is a treeview that I created as part of another article. I won't be focusing much on the details of the treeview, but if you are interested, you will find all the details in my other article. In short the treeview is created in React and uses Flux for state management.
The React component is self contained and packaged up independently, but how can we go about injecting it into the Angular world?
It turns out that Angular components implement an optional onInit() hook that we can take advantage of to trigger the code to render the React component.
In my host component I define an implementation for the onInit handler where I call my static initialize function to kick off the React rendering. As you can see, I have wrapped the ReactComponent in a class that I am importing in the host.
The initialize method is also pretty straightforward, but notice how I am passing in a title text that is passed down to the React component as a prop.
There is a lot of discussion about different JavaScript frameworks like Angular, React, etc these days, but one uniting factor across frameworks seems to be TypeScript. As of TypeScript 1.6 the TypeScript compiler supports jsx out of the box. This is a huge help since it means we can manage both the Angular code and the React code in the same compilation step. In the end JSX is valid TypeScript that gets transpiled down to regular JavaScript. The only thing you have todo differently is tell the compiler that you are using JSX by specifying the jsx flag:
Bellow is a screenshot of the treeview, but you may also test it out by going to my live demo. The code is also available on Github
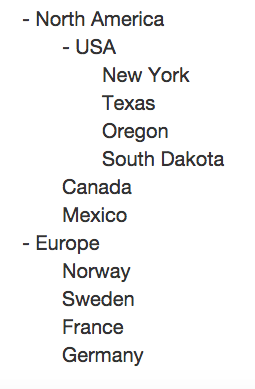