In the following article I will show how to use ComponentFactoryResolver in Angular to insert components dynamically into the DOM
In Angular we typically load a component as a tag in the markup, perhaps assisted by some conditionals or for loops to make the loading more dynamic. Generally this is the recommended approach, but there are cases where you don't know the DOM structure at compile time. In these cases we need to be able to add components on demand in arbitrary locations. It might be tempting to fall back on jQuery for this, but Angular provides a ComponentFactoryResolver that we can use instead.
To demo this feature I have created a graph component where I am letting the user connect vertices with edges to form a graph. I am modeling the edges as separate components, but since the user can connect any pair of nodes, there is no way to know ahead of time where to put the edge components.
I decided to model this using three components; Graph, Vertex and Edge. Graph and Vertex instances are statically placed in the template to form a selection of disconnected nodes. By clicking on two different vertices, an edge is drawn to connect them. This is where ComponentFactoryResolver comes into play by allowing me to load components into the DOM.
The code for the three components looks like the following:
Vertex
Vertex defines a node in the graph and exposes the x,y coordinates of the node in the DOM. When a vertex is clicked, the coordinates are emitted using rxjs via the EdgeService.
Edge
Next up is the Edge component.
Basically the purpose of this component is to draw a line between two sets of coordinates. Part of this involves calculating the angle of the line between the points which can be found using atan2 and some supporting calculations.
Graph
Graph is the container component and the part that ties it all together.
The graph component subscribes to the coordinates from the clicked vertices via an rxjs observable. The stream is set up to buffer and not return anything until two vertices have been clicked.
EdgeService is pretty simple with some buffering logic to ensure we have two sets of coordinates before we send the values off to the subscriber.
ComponentFactoryResolver allows us to locate a component factory by component type, but before we can resolve a factory for the Edge component, we have to tell Angular to generate one by listing Edge as an entryComponent in the current NgModule.
We use the component factory in combination with a ViewContainerRef to load the component into the DOM. coordinates.first.viewContainer is a ViewContainerRef and serves as a reference point for where to place the component. coordinates.first.viewContainer refers to the first vertex we clicked.As you can tell from the screenshot below clicking pairs of nodes will enable you to connect any pair of nodes.
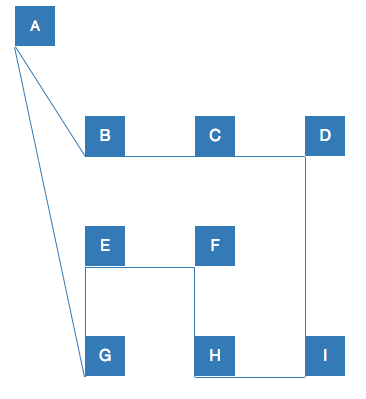
As always the code is available on Github and a live demo is of course also available.