In this post I will examine how Angular applications grow in size when moving beyond the “Hello World” baseline example.
This is important since the size of your JavaScript affects not only download times. It's also increases the time the browser has to spend parsing the incoming JavaScript.
Angular 1.x vs Angular >= 2
Angular 1.4.7 is around 59k after minification/gzip. Including the optional router library ui-router adds another 10k, so you are probably looking at a total of roughly 70k.
Angular 1.x ships as a pre-built “one size fits all” bundle, with no way to customize the bundle based on application needs. This is where the bundling of Angular 1.x and Angular >= 2 differ the most.
Post Angular 1.x the bundling strategy has moved to a strategy where devs can opt-in to only the parts of Angular needed to run their specific application.
The mechanism behind this is called Tree Shaking.
Tree Shaking means walking the code dependency paths of your application, top to bottom. Any unused code can be excluded from the final bundle, leaving you with a potentially much smaller application bundle.
The less you use of the Angular framework, the smaller your bundle will be. At the time of writing the smallest possible Angular application is roughly 48.7k. This is of course nothing more than a “Hello World” app that utilizes just the bare minimum of the Angular framework.
Going Beyond Hello World
The baseline size is pretty impressive, but what happens when you start building a more substantial application?
Tree Shaking is a process of removing unused code. You have to expect to give back some of your size reduction when more of the Angular framework is exercised.
Adding Modules
In the following section I will measure the impact of extending the Hello World application by brining in the different Angular modules one by one.
The samples are intentionally left simple since the point of this experiment is to measure the size impact of the Angular framework itself.
The point here is to provide implementations that force inclusion of enough Angular modules to be realistic in a real world application.
Hello World
The baseline experiment is the Hello World application. This application is of course just of academic interest, but it gives us the lower boundary for the size of an Angular applications

The minifed/gzipped size is 48.7k with the following source map explorer snapshot of the bundle.
If you examine the bundle you will see very few Angular modules included.
FormsModule
Next I have extended the application to include the FormsModule in my application. The FormsModule gives you access to ngModel and Template based forms. Both of these are key features and likely to be needed by most applications.
The application builds a simple form with some basic validation and form submission logic:
After including the FormsModule my bundle size increased to 61.3k. Here is the updated source map explorer snapshot.
ReactiveFormsModule
The FormsModule has a sister module in the ReactiveFormsModule. This module opens the door to ReactiveForms, which represents a different approach to building forms.
Most applications may choose either Template forms or Reactive forms, but it's not unlikely for a large application to benefit from both.
I extended the application to include a Reactive form as well:
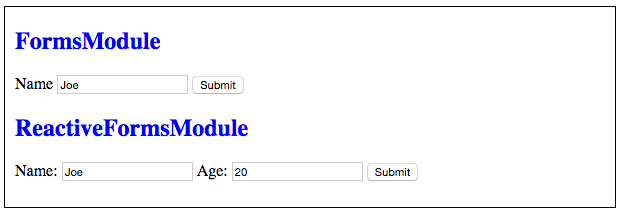
The impact of adding the ReactiveForms module is barely noticeable in the bundle size. The new size is 62.6k, which is only 1.3k bigger.
Here is the updated source map explorer snapshot.
HttpModule
Next I will examine the impact of adding the http module.
In this implementation I've added three very common patterns of http requests: Single request, chained requests and parallel requests.
Angular has decided to move in the direction of an RxJs based http implementation. This means we also have include a few RxJs operators to support the http module (flatMap, forkJoin and map).
After adding the three types of http request my application looks like this:
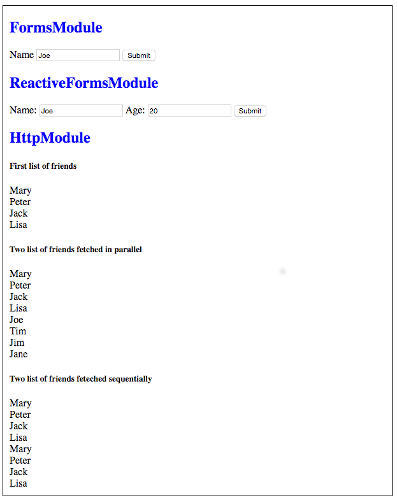
The new size of my bundle is 71.3k with the following source map explorer snapshot.
RouterModule
Finally I will add the RouterModule with a basic routing implementation. Routing can be made much more complicated, but here I am sticking to a few root level routes with a single parameter.
Clicking one of the links loads a simple component that displays the name of a color.
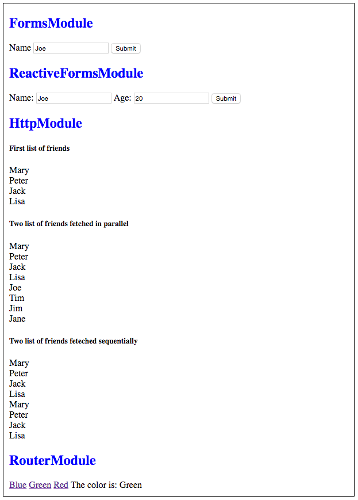
After including the RouterModule my bundle size increased to 91.2k.
The updated source map explorer snapshot can be found here.
New Baseline
The bundle size will of course vary based on application needs. I find 90k+ to be a realistic Angular footprint though. Not all applications will need to include all modules, but a decent size enterprise application likely will.
All the bundle sizes reported so far include only the size of Angular + some minor application code. In addition to this we also have 26.5k from core-js and 16.1k from zone.js. These dependencies are necessary for the Angular application to run, so they have to be counted towards the final footprint.
This puts us at a new baseline of roughly 134k.
This is of course quite a bit larger than the footprint of Angular 1.x, but still pretty good all things considered. It's also likely that the footprint will shrink by adding the Closure compiler to the build process.
For more detail I have an article here explaining why the Closure compiler may be more effective than standard Tree Shaking.
I have put the source code for this experiment of Github.