Most Angular developers are probably already familiar with the $timeout service. This service serves an important role in Angular 1.x, so today I decided to go digging for its counterpart in the new Angular world.
As you may know, $timeout is essentially a wrapper for JavaScript's native setTimeout function. At first glance it almost seems ridiculous to wrap setTimeout with an almost identical feature, but there are good reasons for this wrapping. Basically it all comes down to Angular's implementation of dirty checking and providing a way to inform Angular that your object updates should be enlisted in a digest cycle. If you update your objects in a plain setTimeout call there is no way for Angular 1.x to know to update your view based on your changes – unless you trigger a manual digest cycle. This is where $timeout comes into play by automatically enlisting the updates in a digest cycle.
Now, how does this work in post 1.x Angular?
I initially thought the approach would be very similar. I stumbled upon a class called TimerWrapper that I thought would be the answer to this. However, after tweeting to Angular member Viktor Savkin I learned that TimerWrapper is part of the internal API. He also told me we no longer need to worry about kicking off digest cycles manually when dealing with window.setTimeout. Instead zone.js will do it for us, which means we can go back to using regular window.setTimeout. I will have to learn more about zone.js at a later time since this is a new concept to me.
Insertion Sort
To have some fun with setTimeout I decided to use it to animate an implementation of the insertion sort algorithm. Insertion sort is one of the simple algorithms you may remember from your CS data structures curriculum, but if you don't, that's ok for the purposes of this article :-)
The idea is to use setTimeout to delay object updates as the algorithm shuffles through the array as the sort executes. As an element is shuffled I give it a different background color until the next element is shuffled. As always I have a live demo of this, but the UI looks something like this:
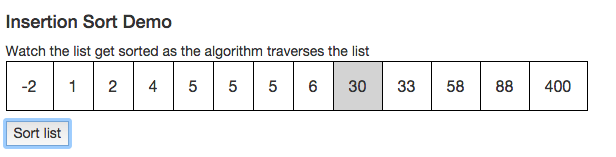
I won't cover the details of Insertion sort here, but in the source code below you can see how I have integrated setTimeout in the shuffling stage of the algorithm.
window.setTimeout() takes a lambda expression and a timeout in milliseconds representing the execution delay.