In this post I will show how to integrate Firebase with an Angular application.
I will show how to use Firebase as a realtime database with data synchronization between connected clients.
For the purposes of this article I will create a simple “Friends” application.
Users can use the application to create a list of friends. The list is synched between all active browser sessions. Additions and removals are pushed to all users realtime, so if you have multiple browser windows open, changes will appear in all browser windows.
The Friends application is created using Angular CLI. I have also added AngularFire2 to simplify the integration between Angular and Firebase.
Create the database
Before we can connect to Firebase we have to create our own Firebase instance using the Firebase web console. The process is very simple, just follow the directions in the Firebase admin UI.
Once the database is created you can use the admin UI to specify access permissions for the database instance. There are several options to choose from (Twitter, Facebook, Github, etc).
For this demo I've kept it simple and disabled authentication. This is just for demo purposes. In a real application you should require some sort of user authentication.
The schema of the database is json based. You can use the admin UI to upload a json file to seed your database with some data.
Accessing the database
I now have a publicly available database hosted in the Firebase cloud, but I need some sort of connection string to access the database from the Angular application.
The connection string can be exported from the admin UI.
Here is the format:
Just substitute the values in []'s with the values specific to your own database.
Create the Angular application
Now that I have a connection string I can use it to configure the Friends application to talk to Firebase.
app.module.ts
The connection string is passed to the AngularFireModule.
AngularFire is downloaded separately from npm by running
Next I will create a FriendService to talk to Firebase
friend-service.ts
The service offers some basic crud methods to manage the list of friends. The data is stored in a list called "friends". This list was initially populated through the admin UI when I uploaded my seed json file.
The only remaining part is creating a simple component with a UI to display, add or remove friends.
app.component.ts
app.component.html
Here is a screenshot of the application:
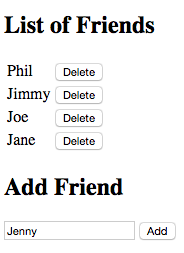
I have deployed the code to Github if you are interested in trying it out.